Lab 1
Understand Lab Policy:
- Structure: Labs will consist of a working through a problem set or going over relevent lecture material.
- Attendance: You will have to submit a form with a secret along with your BU username in order to be marked present. The secret will be given out towards the end of lab or when you finish the assigned task.
- Collaboration: You are encouraged to work with your classmates on the exercises in lab.
Creating a new Java project
In Lab 0 you should have set up Eclipse, which is the environment that we’re recommending for your work in CS 112. Lets create a Hello World application together!
- Open up Eclipse and click Launch
- Set up a new project. We can use the same project for all the labs in this class.
- Select Java project and click next
- Name your project "labs" and click finish!
- Click open perspective if prompted
- You should now see your project on the side bar and be able to expand it as follows. Select the folder src.
- With src highlighted create a new class as follows:
- Name your new class, make sure to select the first stub (see below) and ensure that the name is lab1, and click finish
- Congradulation we've set up our Java file! You should now see this:
- Try to add code in the main section of the code and run the program and get the console to display
HelloWorld
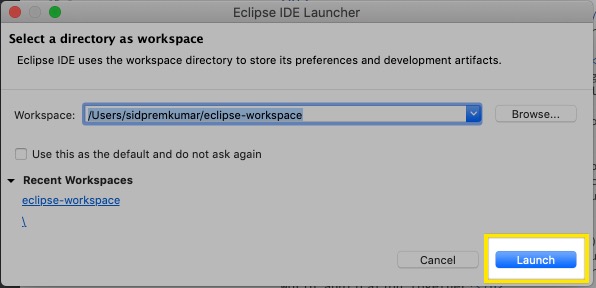
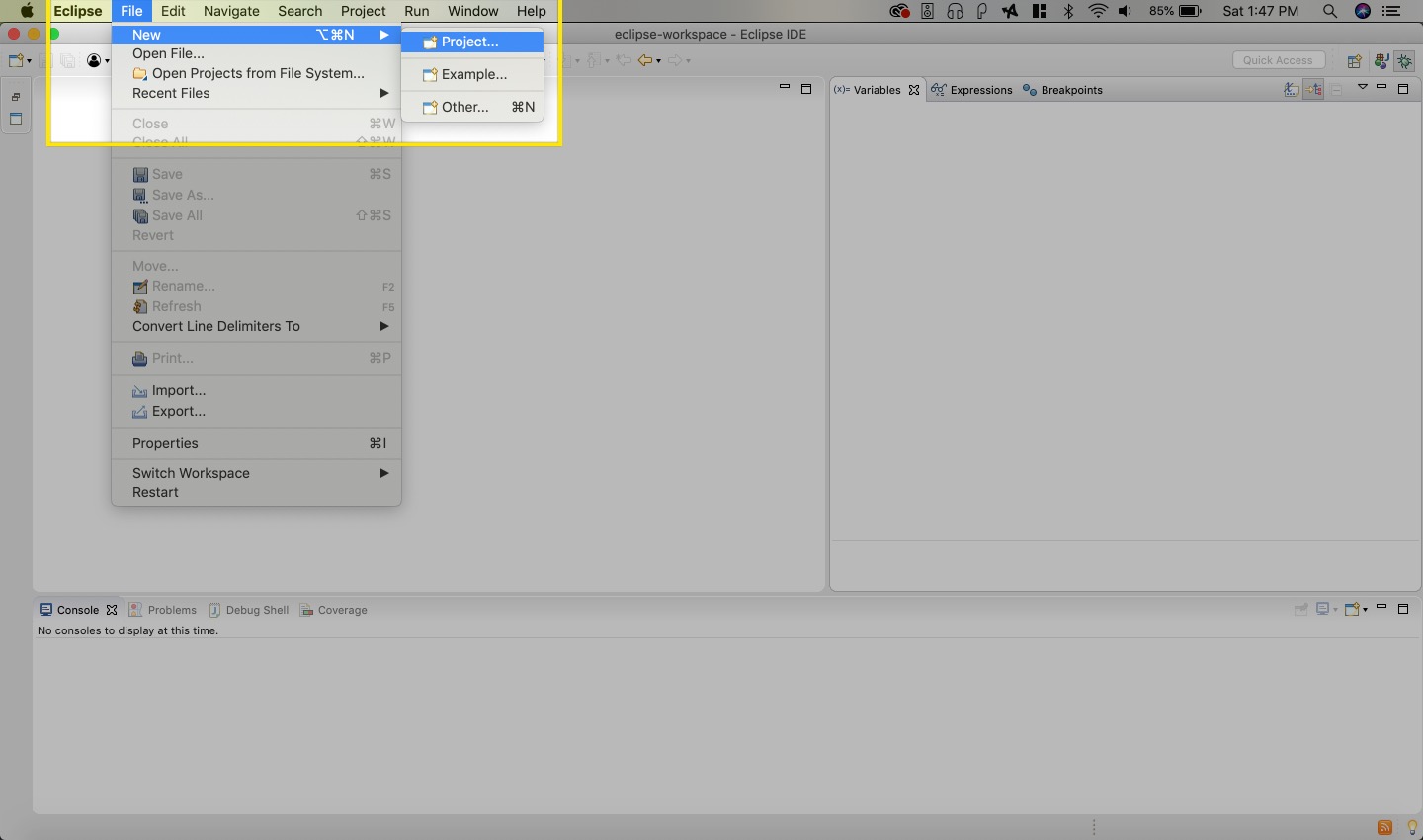
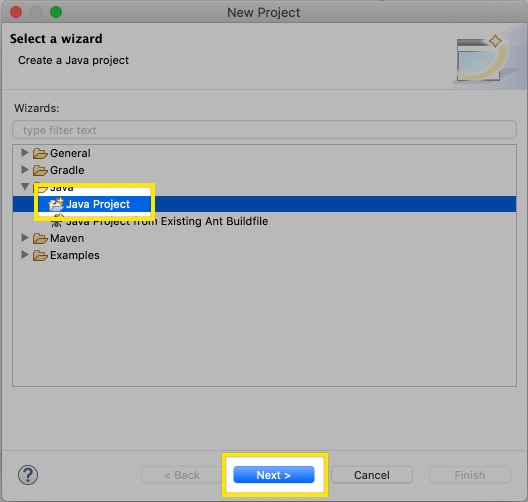
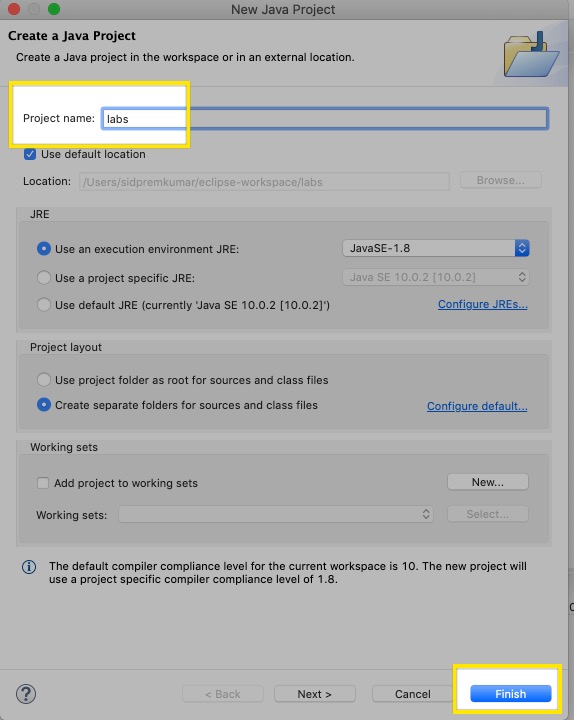
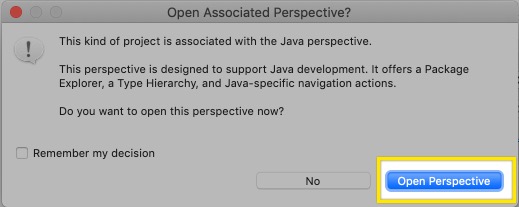
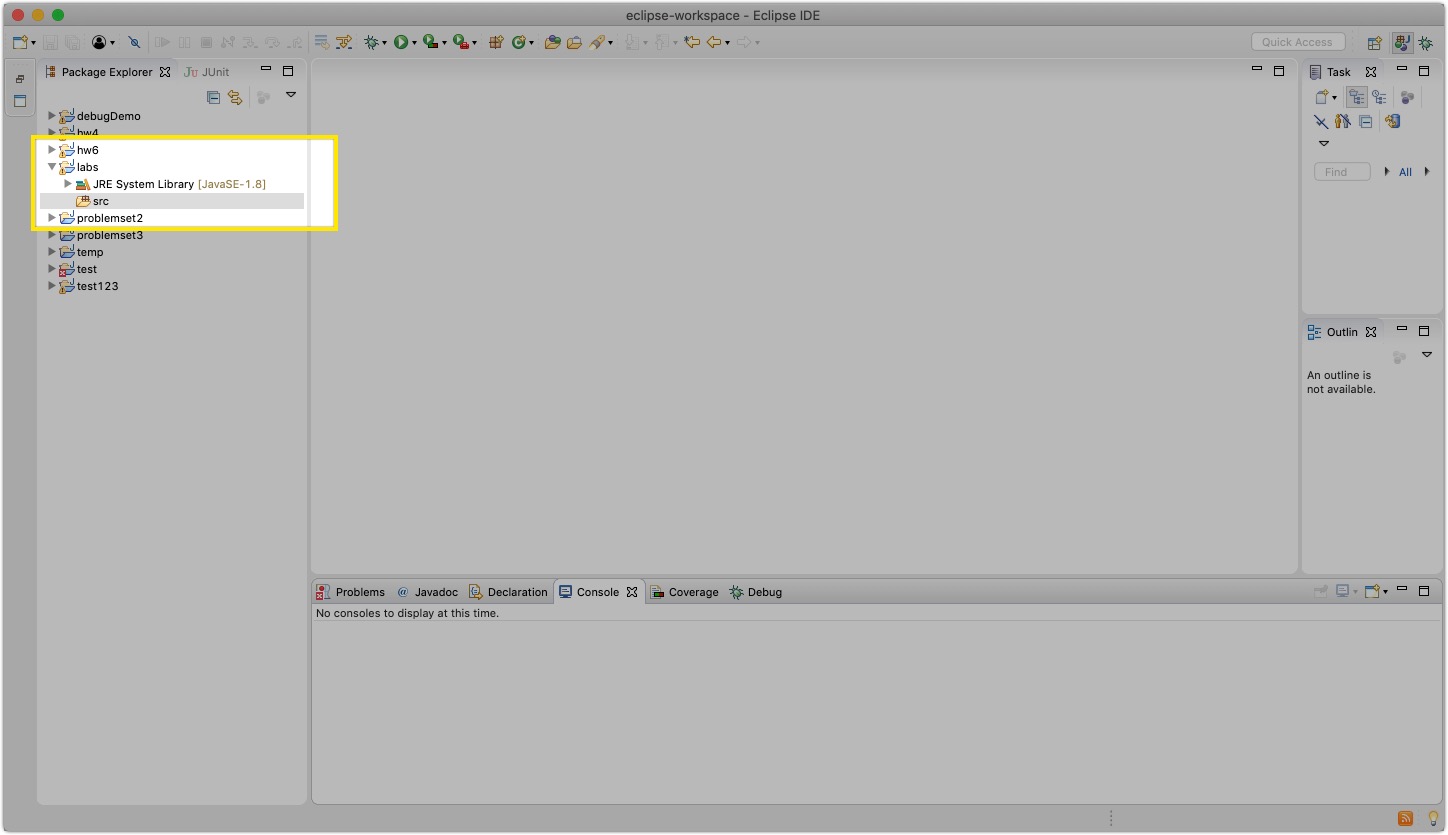
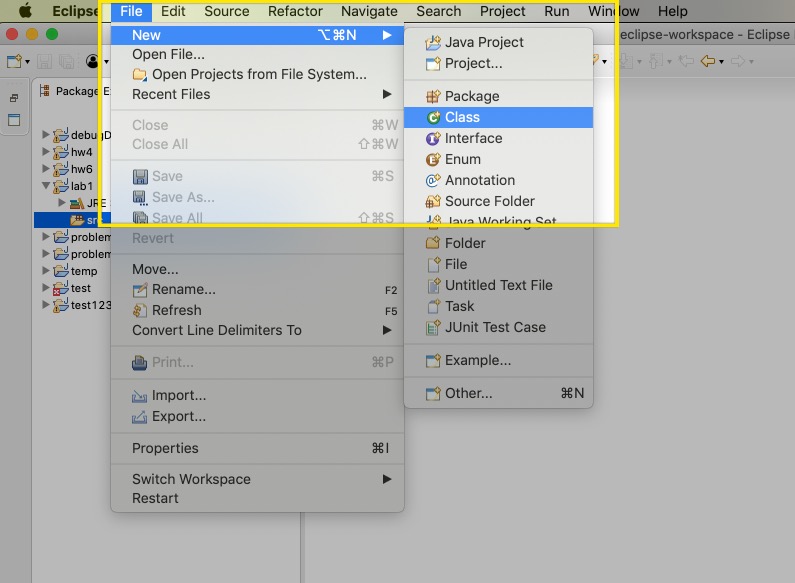
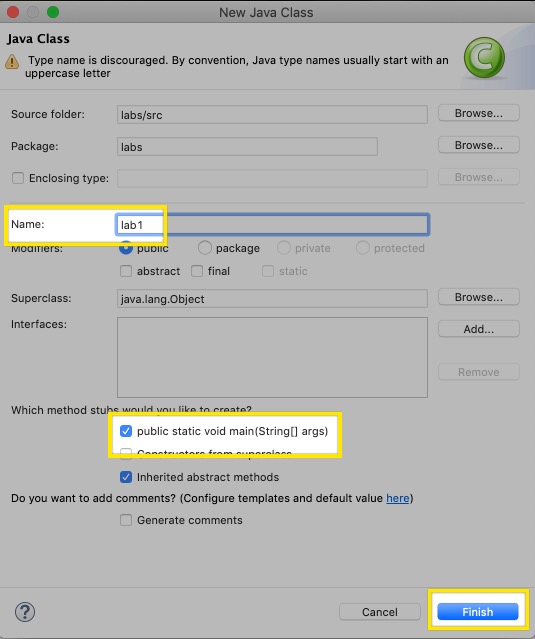
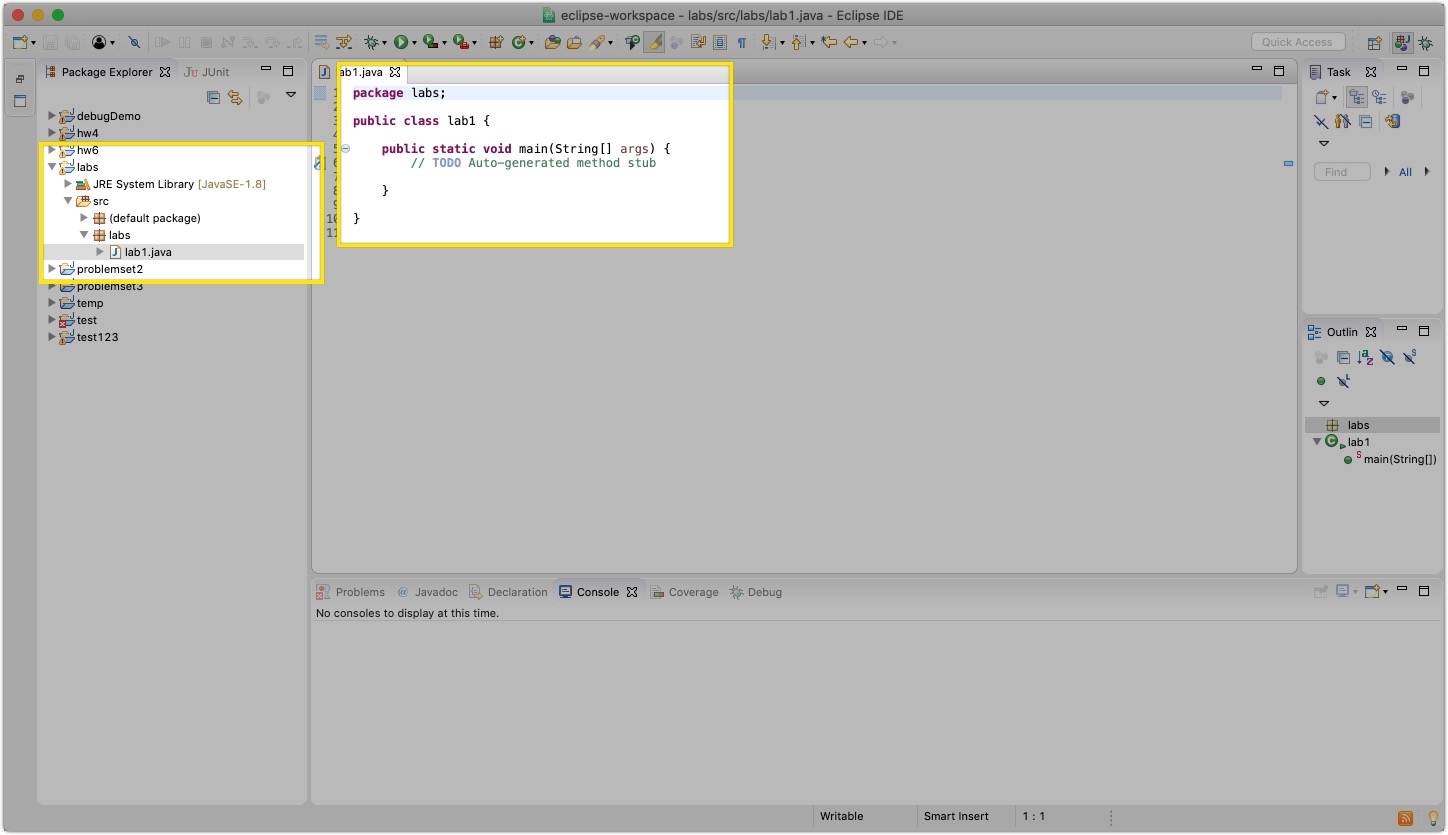
Functions
Copy the following code into the main section of your code:
int [] a = {2, 5, -2, 6, -3, 8, 0, -7, -9, 4};
int target = 0;
for (int i = 0; i < a.length; i++ ) {
if(a[i] == target) {
System.out.println("Element found at index " + i);
break;
}
}
Your class should look like this:
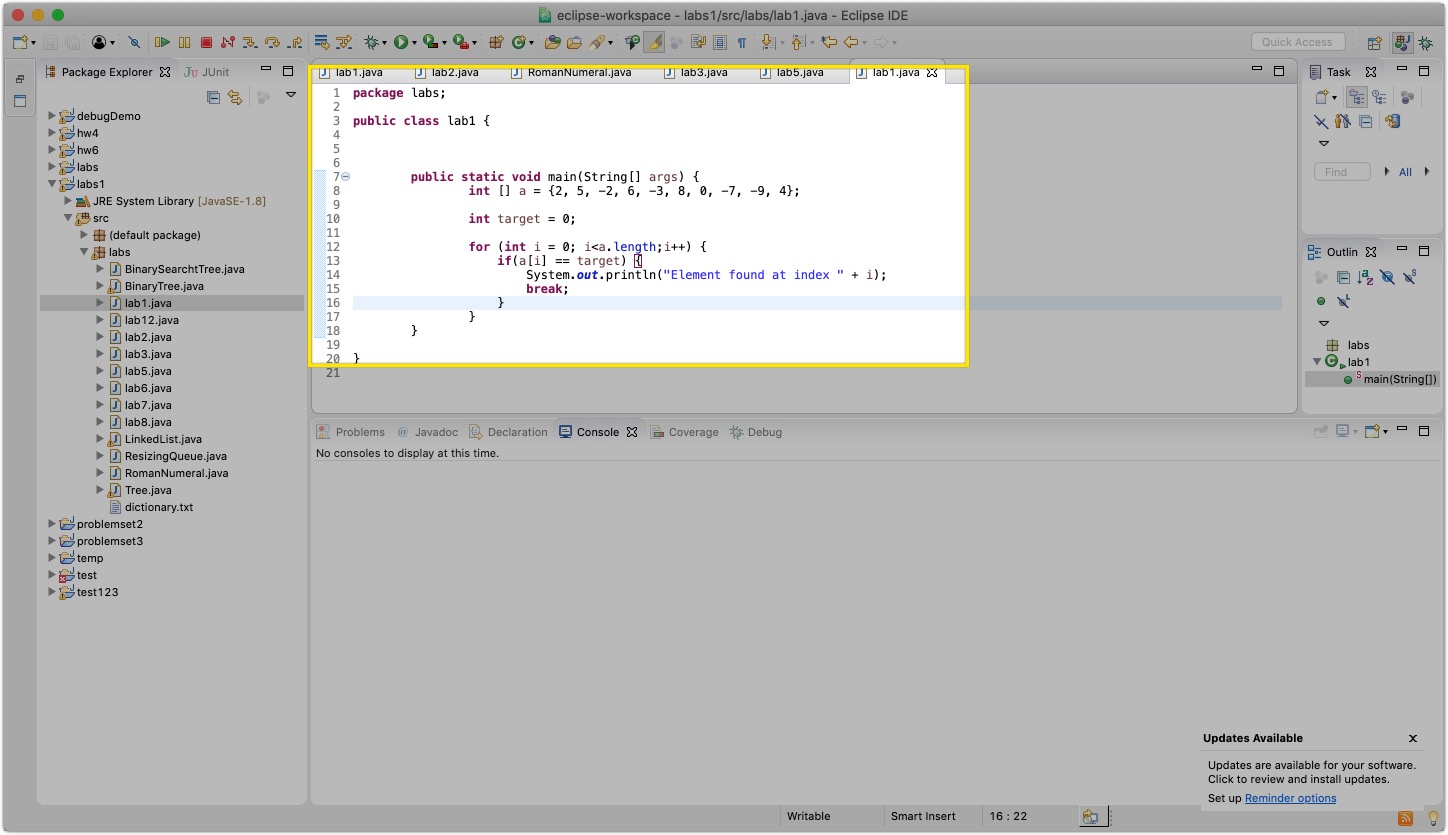
Try running the code and you should get this as an output:
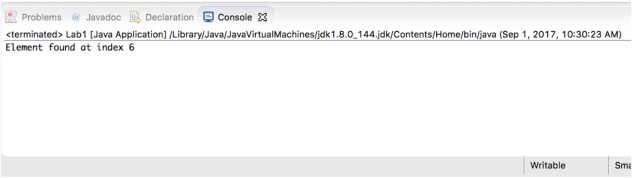
Copy the folowing code into your class (Note: we are replacing the main function)
public static void main(String[] args) {
int [] a = {2, 5, -2, 6, -3, 8, 0, -7, -9, 4};
int target = 0;
performSearch(a, target);
}
public static void performSearch(int[] a, int target) {
}
Your Eclipse should look like this now:
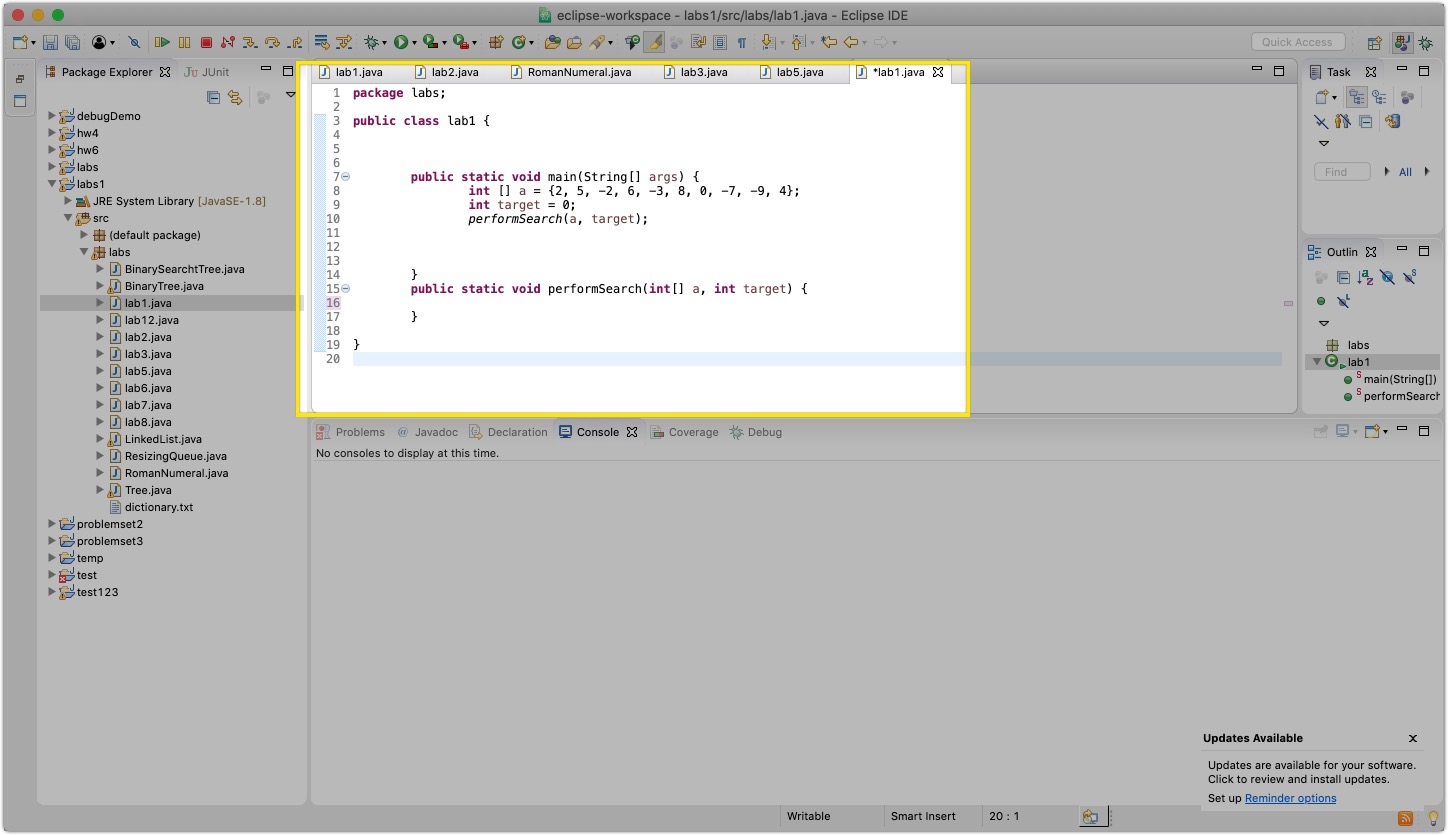
Modify the code so that you create a function as follows: public static void performSearch(int[] a, int target)
. Let us understand what this all means:
void
: void means that the output of the function is nothing. i.e., the function does not return anything.performSearch
: This is the name of the function.int[] a
: This is the first input into the function. This is an array of ints.int target
: This is the second input into the function. This is simply a single integer.
We ask you to complete the function performSearch
so that Lab1 can compile and run and give you back a similar output as seen in the previous figure.
Debugging Exercise
Copy the folowing code inside the public class lab1 {
in the project we just created (Note: we want to also replace the main function for this lab):
public static double triArea(int b, h) {
area = b/2*h;
return area
}
public static void main(String[] args) {
System.out.printf("Running lab1.java\n");
double a = lab1.triArea(5,3);
System.out.println("Area is: " + a);
}
You'll notice that there are some errors in the code. Eclipse does a great job of showing you where they are:
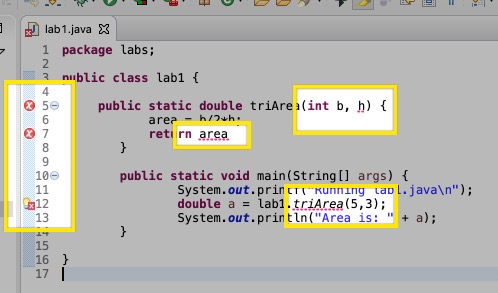
Take a couple of minutes to try and figure out the errors. In a few minutes we'll go over them as a class.
To runm the program click the run button. If you debugged correctly you should see a 6.0:
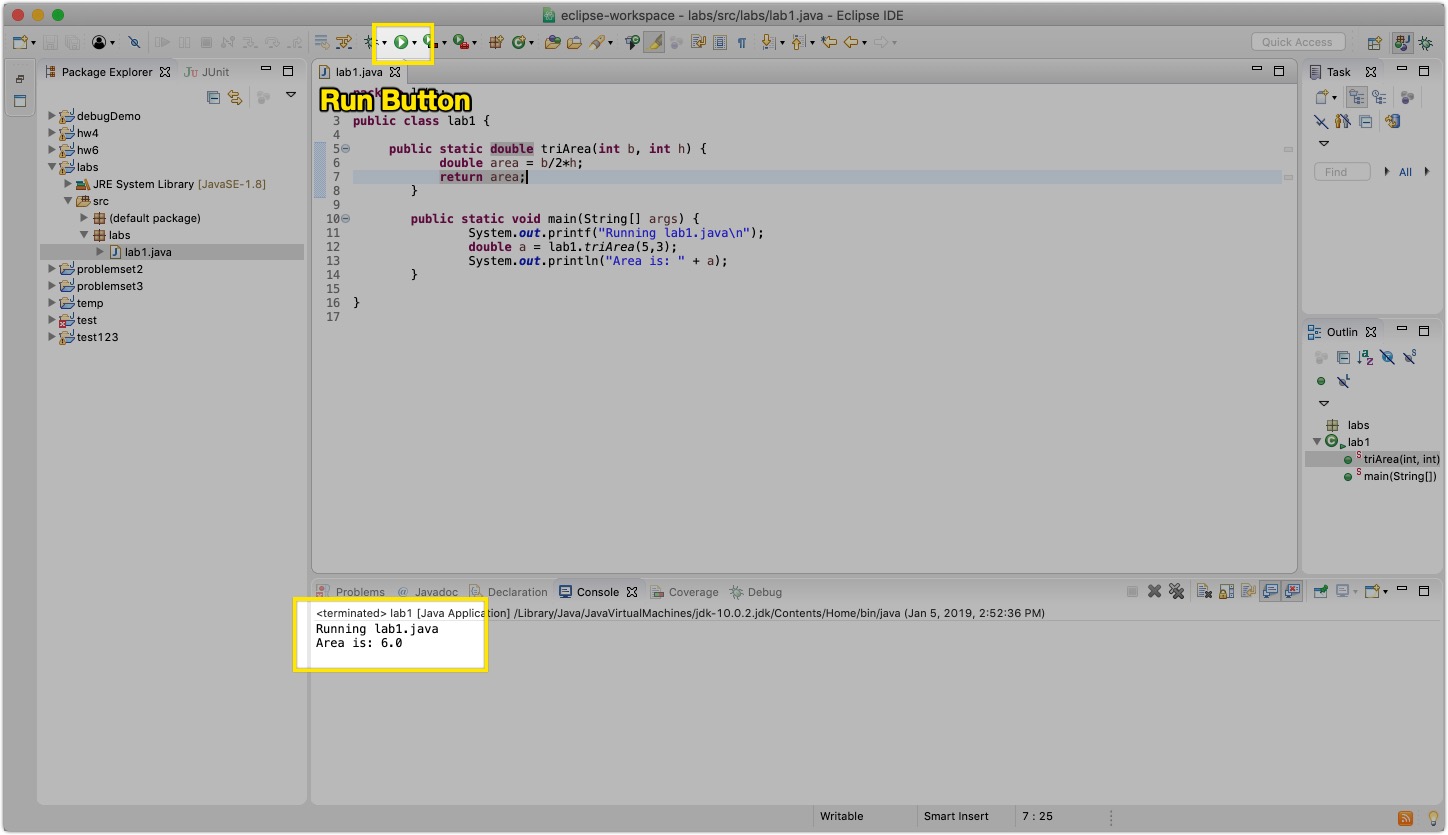
Java String Exercise
Before we start this exercise take a look at this helpful cheat sheet:
Operation | In Python | In Java |
---|---|---|
assigning to a variable (and, in Java, declaring the variable) | s1 = 'Boston' s2 = "University" |
String s1 = "Boston"; String s2 = "University"; |
concatenation | s1 + s2 | s1 + s2 |
finding the number of characters | len(s1) | s1.length() |
indexing (note: Java does not have negative indices) | s1[0] s2[-1] |
s1.charAt(0) s2.charAt(s2.length()-1) |
slicing | s1[1:4] s2[3: ] s1[ :5] |
s1.substring(1, 4) s2.substring(3) s1.substring(0, 5) |
finding the number of characters | len(s1) | s1.length() |
converting all letters to uppercase | s1.upper() | s1.toUpperCase() |
converting all letters to lowercase | s2.lower() | s2.toLowerCase() |
determining if a substring is in a string | s2 in s1 'sit' in s2 |
s1.contains(s2) s2.contains("sit") |
finding the index of the first occurence of a character or substring | s1.find("o") s2.find('ver') |
s1.indexOf('o') s2.indexOf("ver") |
testing if two strings are equivalent | s1 == s2 s1 == 'Boston' |
s1.equals(s2) s1.equals("Boston") |
Now to finish the lab, mordify the project you created in order to produce this as an output:
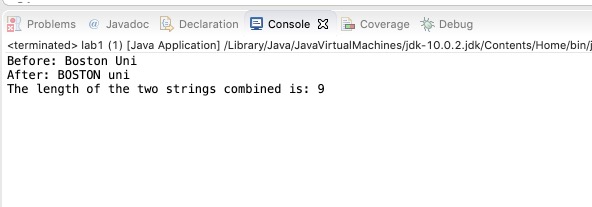
Make sure to mark your attendance here before leaving!